Introduction
Managing complex forms can be a daunting task, especially when the form requires multiple steps to complete. React’s single-form approach often leads to cumbersome code and poor user experience. Multi-step forms break down the process into manageable chunks, enhancing usability and keeping users engaged. They simplify validation, improve organization, and offer a more structured approach to collecting extensive data. By implementing multi-step forms, developers can create a more intuitive and efficient user experience, making complex data entry tasks feel less overwhelming and more organized.
Prerequisites
- Node.js and npm
- React
- Typescript
- React hook form
Navigating Complex Forms : The Case for Multi-Step Forms in React
Why Multi-Step Forms Matter
Building forms with React can quickly become a challenge, especially when dealing with complex, multi-step processes. Traditional form handling can be tedious, involving verbose setup for state management, validation, and submission. This complexity grows with each step you add, making it easy to feel overwhelmed by the task.
The Need for Multi-Step Forms
Multi-step forms address this by breaking down complex input processes into manageable stages. This approach improves user experience by guiding users through the form in a structured manner, reducing cognitive load, and enhancing form completion rates. However, implementing multi-step forms requires careful planning and effective state management to ensure a seamless flow.
Simplifying Multi-Step Forms with React
Leveraging React’s component-based architecture and state management capabilities, you can create efficient multi-step forms that are both user-friendly and maintainable. In this blog post, we will explore strategies for building multi-step forms in React, focusing on state handling, dynamic rendering, and user navigation. By the end, you’ll have a clear understanding of how to implement multi-step forms effectively, improving both user experience and development efficiency.

Navigating the Multi-Step Form Setup in React
Creating a seamless user experience often requires breaking down lengthy forms into manageable segments. In this guide, we will explore the implementation of a multi-step form using React and TypeScript. By leveraging React Hook Form, we’ll simplify form state management and validation across multiple steps. This approach not only enhances user experience by guiding users through the form incrementally but also maintains clarity and efficiency in data handling. We’ll walk through the setup and organization of each step, demonstrating how to create a fluid and intuitive form submission process.
Initializing React Application : To create a React application, you need to start by creating a project folder in your root directory.
//Create react app using the following command
npx create-react-app multistep-form --template typescript
//Install the required library
npm i react-hook-form
npm install @mui/material
npm install @emotion/react @emotion/styled
Setting Up Project
- Project Structure : Within your project directory, locate your App.tsx file which acts as the main entry point for your React application.
- Creating Components :
- Create a Components Folder : Inside your project, create a folder named components to organize your reusable components.
- Create MultiStepController.tsx : This component will handle the overall management of form steps and state transitions, encapsulating the logic required for navigating between steps and processing form data.
- Add Files for Multi-Step Form
- MultistepForm.tsx : This file will contain the React component responsible for rendering the multi-step form. It manages the form state, handles step transitions, and integrates with the MultiStepController for managing the multi-step logic.
- ReusableTextField.tsx : This component provides a reusable text field with integrated validation using React Hook Form. It simplifies form field management across different steps.
// App.tsx
import MultiStepFormController from "./components/MultiStepFormController";
const App = () = > {
return ( div>);
};
export default App;
In the App component, we are rendering the MultiStepController component, which is a key part of our application’s interface for managing multi-step form functionality.
//MultiStepFormController.tsx
export interface IFormData {
firstName?: string;
lastName?: string;
age?: number | null;
email?: string;
contactNo?: number | null;
city?: string;
country?: string;
zipCode?: number | null;
}
const initialFormData: IFormData = {
firstName: "",
lastName: "",
age: 0,
email: "",
contactNo: 0,
city: "",
country: "",
zipCode: 0,
};
const MultiStepFormController = () => {
const [formData, setFormData] = useState(initialFormData);
const [step, setStep] = useState(1);
let currentContent: JSX.Element | undefined;
switch (step) {
case 1:
currentContent = (
);
break;
case 2:
currentContent = (
);
break;
case 3:
currentContent = (
);
break;
default:
currentContent = (
Your details have been submitted successfully!
//Rendering Submitted Form Data
);
}
return <>{currentContent}>;
};
export default MultiStepFormController;
The MultiStepFormController component manages the multi-step form process by controlling the current step and form data. Here’s a brief overview of its key functions:
- State Management
- formData : Holds the current values of the form fields, initialized with default values in initialFormData.
- step : Tracks the current step of the multi-step form, starting at step 1.
- Content Rendering
- currentContent : A variable that holds the JSX element to be rendered based on the current step. It uses a switch statement to determine which MultiStepForm component to display.
- Form Submission
- In the default case of the switch statement, after all steps are completed, the form data is displayed in a summary format. This summary shows all the collected data from the previous steps.
- Return Statement
- The component returns currentContent, which will be the form for the current step or the summary view, based on the step state.
Overall, the MultiStepFormController component orchestrates the multi-step form process, handling the transitions between steps and managing form data.
//MultistepForm.tsx
interface IMultistepForm {
formData: IFormData;
setFormData: React.Dispatch>;
currentStep: number;
setStep: React.Dispatch>;
}
const steps = [
"Personal Information",
"Contact Information",
"Location Details",
];
const MultistepForm: React.FC = ({
formData,
setFormData,
currentStep,
setStep,
}) => {
const {
handleSubmit,
control,
formState: { errors },
getValues,
} = useForm({
defaultValues: formData,
});
const handleNextStep = (data: IFormData) => {
setFormData((prevData) => ({ ...prevData, ...data }));
setStep((step) => step + 1);
};
const handleBackStep = () => {
setStep((step) => step - 1);
};
const onSubmit = (data: IFormData) => {
setStep((step) => step + 1);
setFormData((prevData) => ({ ...prevData, ...data }));
console.log("Final Data Submitted:", getValues());
};
return (
{steps.map((label, index) => (
{label}
))}
);
};
export default MultistepForm;
The MultiStepForm component handles the step-by-step navigation of the form process.
Step Handling
- currentStep controls which section of the form is displayed.
- The form has three steps:
- Step 1 : Personal Information (First Name, Last Name, Age)
- Step 2 : Contact Information (Email, Contact No)
- Step 3 : Location Details (City, Country, Zip Code)
Step Transitions
- handleNextStep : Updates the form data and proceeds to the next step.
- handleBackStepb : Allows users to navigate back to the previous step.
Form Completion
- On Step 3, the “Next” button changes to “Submit.”
- Once submitted, the final data is captured and processed.
This setup efficiently manages step navigation, ensuring a smooth flow through the form.
//ReusableTextField.tsx
interface ControlledTextFieldProps {
name: string;
control: Control;
label: string;
rules?: object;
type?: string;
}
const ReusableTextField: React.FC<
ControlledTextFieldProps & TextFieldProps
> = ({ name, control, label, rules, type, ...rest }) => {
return (
(
)}
/>
);
};
export default ReusableTextField;
The ReusableTextField component integrates a TextField with react-hook-form for validation and input handling. It renders a controlled text field with custom styling:
- Props
- name, control, label, rules, and optional type to configure the field.
- Functionality
- Uses Controller from react-hook-form to manage field state.
- Displays validation errors via helperText when rules are violated.
This setup ensures reusable form fields with validation and consistent styling.
Output

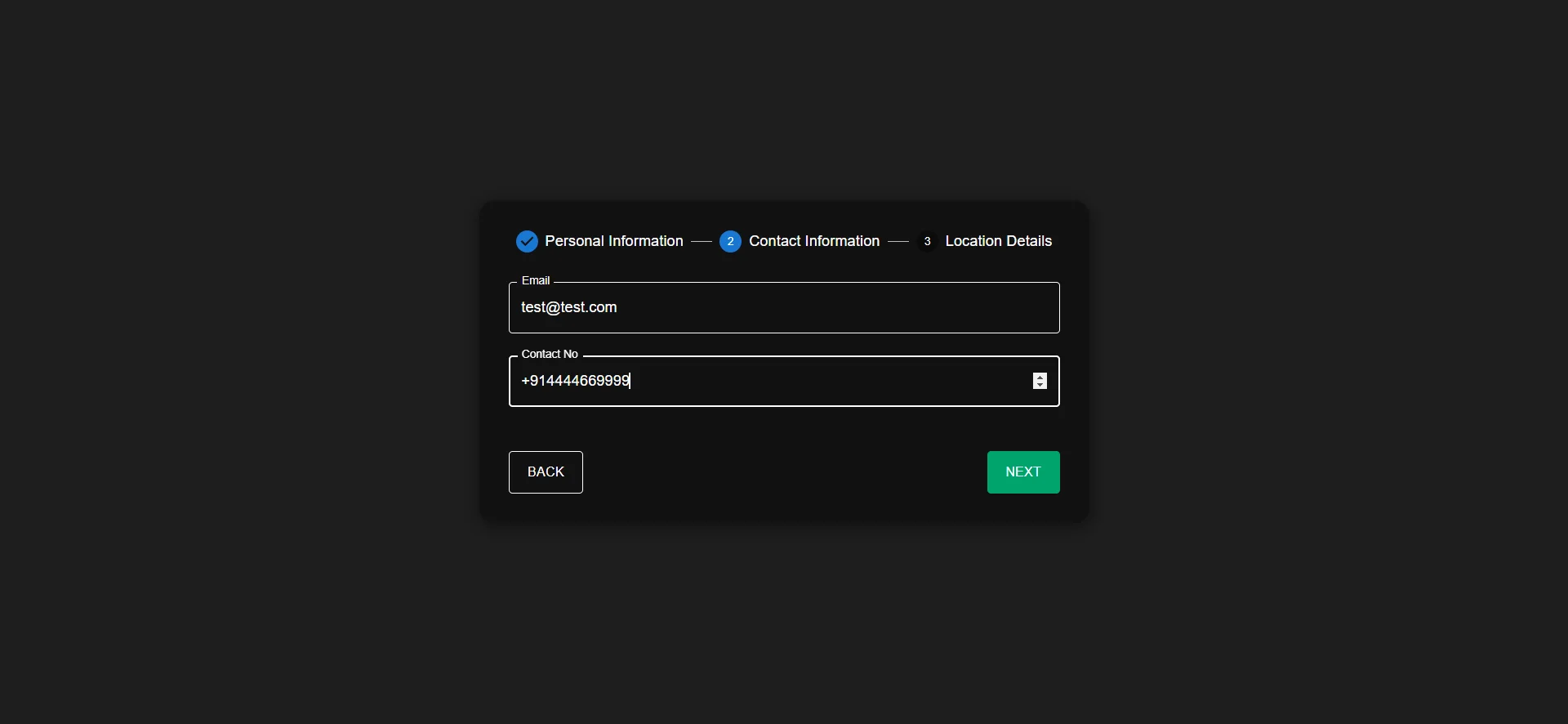
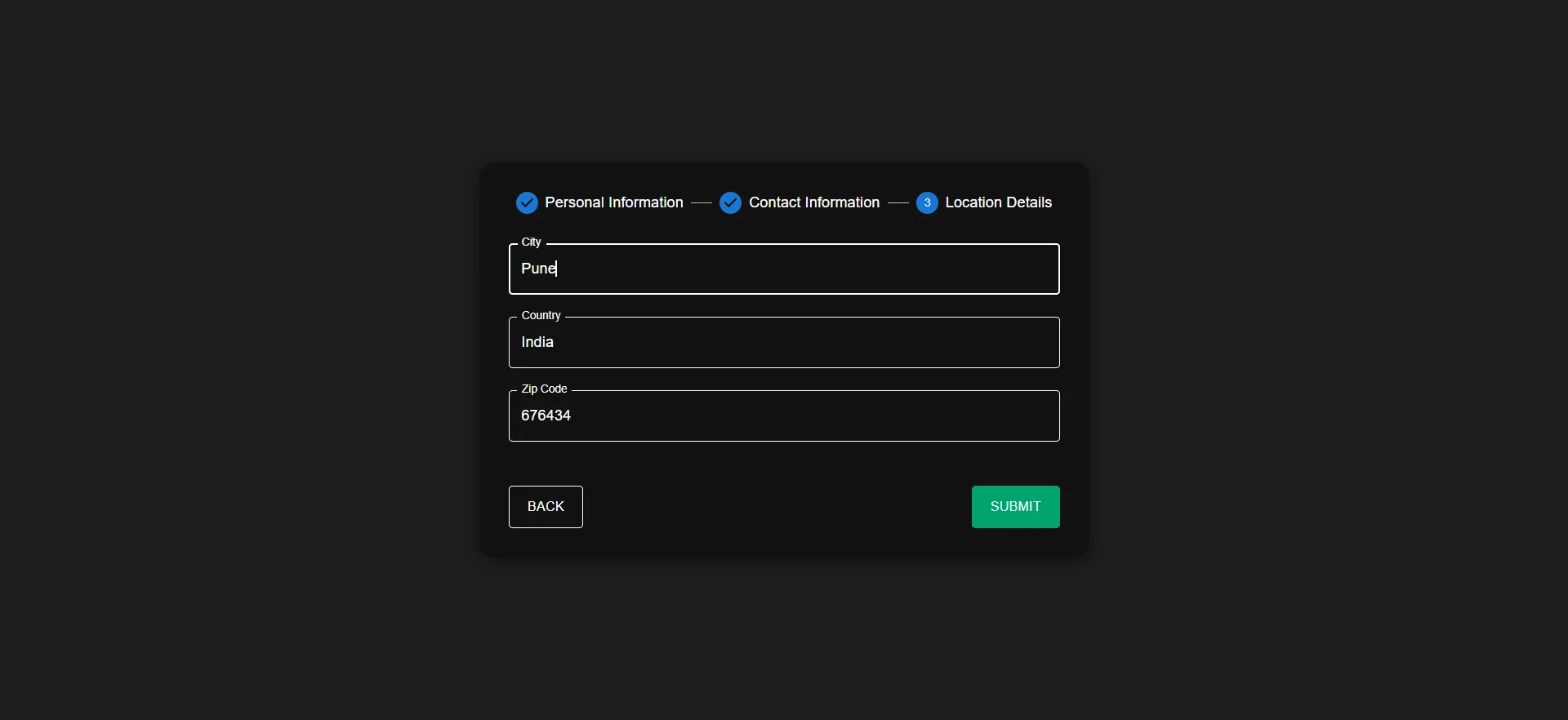

Conclusion
In summary, a multi-step form enhances user experience by breaking down complex forms into more manageable sections. This approach not only simplifies the completion process for users but also makes the form easier to navigate and less overwhelming. Clear, logical progression between steps and a well-designed interface are key to maintaining a smooth and engaging user experience.
Explore Our Services
Discover how we can help your business thrive, whether you’re running a small startup, an SME, or a large enterprise. We’re here to understand your unique needs and goals, offering the expertise and resources to support your journey to success.
Stay informed about our ReactJS services and updates by subscribing to our newsletter—just fill in the details below to subscribe.